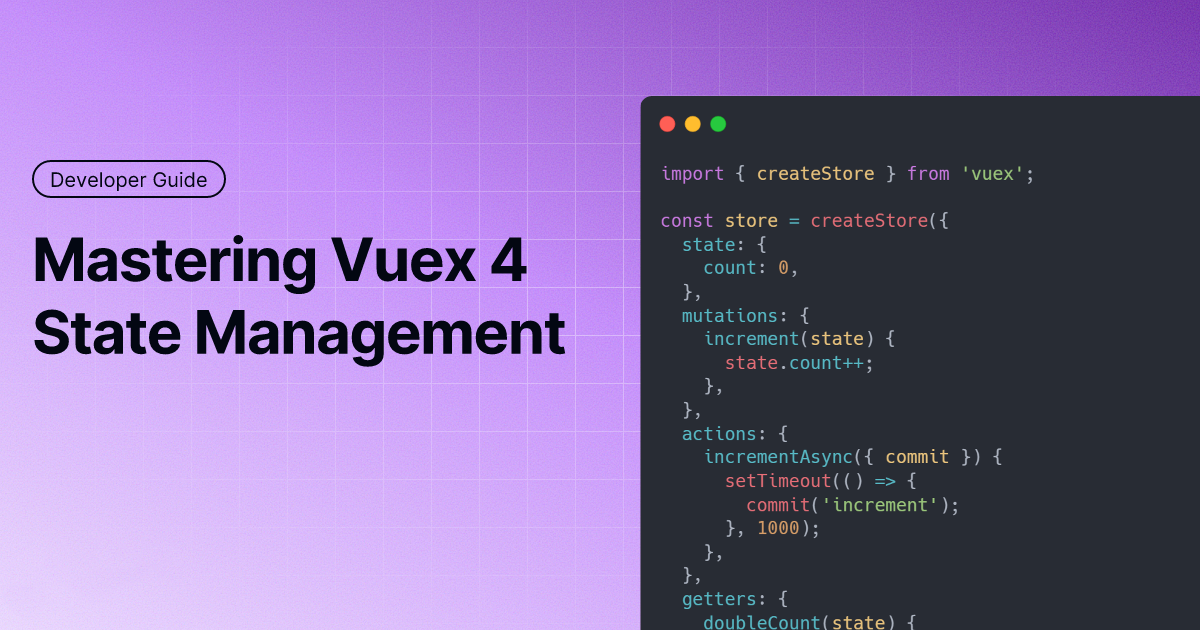
DEVELOPER GUIDEMastering Vuex 4: State Management Strategies for Large-Scale Vue.js Applications
Introduction:
Vuex is a state management library for Vue.js applications, designed to manage the state of your application in a centralized and predictable manner. With the release of Vuex 4, developers gain access to new features and enhancements that streamline state management, especially in large-scale Vue.js projects. This blog post aims to delve into mastering Vuex 4 by exploring advanced state management strategies and providing code examples to illustrate best practices.
- Centralized State Management with Vuex:
Vuex follows a centralized state management pattern, where the entire state of your Vue.js application is stored in a single state tree. This centralization facilitates predictable state management, enables state sharing between components, and simplifies data flow across your application. Here's an example of setting up a Vuex store in Vue 3:
import { createStore } from 'vuex';
const store = createStore({
state: {
count: 0,
},
mutations: {
increment(state) {
state.count++;
},
},
actions: {
incrementAsync({ commit }) {
setTimeout(() => {
commit('increment');
}, 1000);
},
},
getters: {
doubleCount(state) {
return state.count * 2;
},
},
});
export default store;
- Module-based State Organization:
In large-scale applications, organizing state can become complex. Vuex 4 allows you to divide your store into modules, each handling a specific domain of your application's state. This modular approach improves maintainability, scalability, and code organization. Here's an example of module-based state organization in Vuex:
// modules/cart.js
export default {
state: {
items: [],
},
mutations: {
addToCart(state, payload) {
state.items.push(payload);
},
},
actions: {
addToCartAsync({ commit }, payload) {
setTimeout(() => {
commit('addToCart', payload);
}, 500);
},
},
getters: {
cartItemCount(state) {
return state.items.length;
},
},
};
- Using Actions for Asynchronous Operations:
Vuex actions are responsible for handling asynchronous operations, such as API calls or timeouts, and committing mutations to modify the state. By separating asynchronous logic into actions, you ensure that your state mutations remain pure and predictable. Here's an example of an action in Vuex:
// Example action in a Vuex store
actions: {
fetchData({ commit }) {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
commit('setData', data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
},
},
- Getters for Computed State Properties:
Vuex getters allow you to compute derived state properties based on the current state. They are useful for transforming or filtering state data without modifying the original state. Getters are cached and only re-evaluated when their dependencies change, ensuring efficient performance. Here's an example of using a getter in Vuex:
// Example getter in a Vuex store
getters: {
totalPrice(state) {
return state.items.reduce((total, item) => total + item.price, 0);
},
},
Conclusion:
Mastering Vuex 4 is essential for effectively managing state in large-scale Vue.js applications. By leveraging Vuex's centralized state management, module-based organization, actions for asynchronous operations, and getters for computed state properties, you can build robust and maintainable applications with predictable data flow. Incorporate these state management strategies into your Vue.js projects and explore Vuex's advanced features to optimize your development workflow and enhance application scalability.