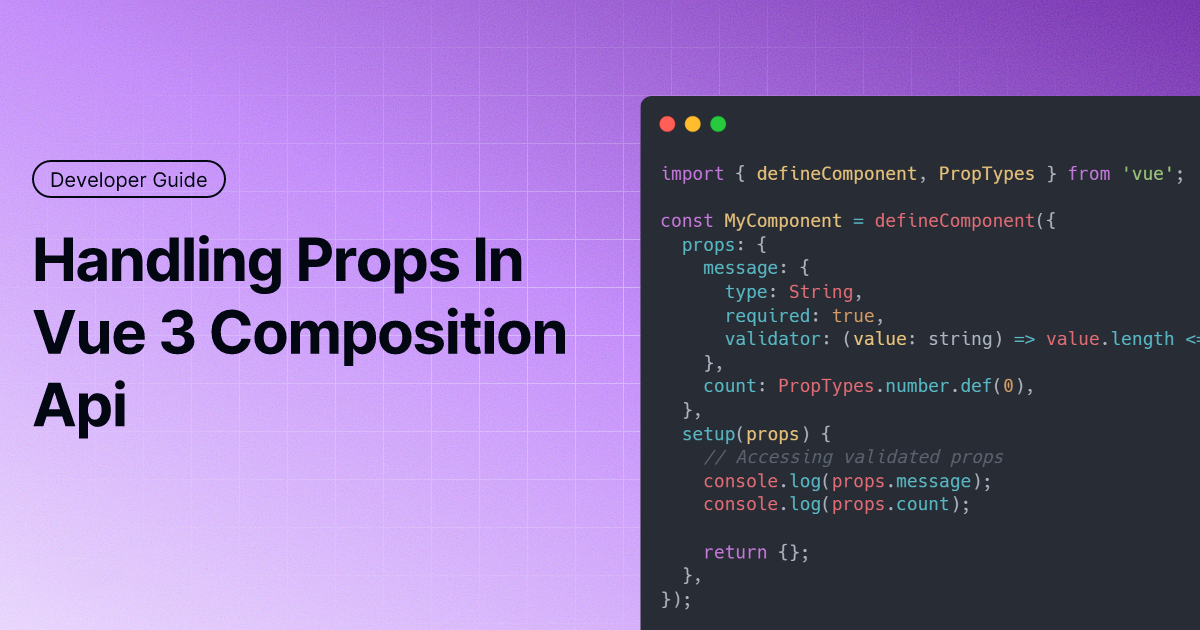
DEVELOPER GUIDEHandling Props in Vue 3 Composition API
Introduction:
Vue.js 3 brought with it the Composition API, a powerful new way to organize and reuse logic in Vue components. One of the key aspects of building robust Vue components is understanding how to handle props effectively. In this blog post, we'll dive into the best practices for handling props in Vue 3 Composition APIs, exploring techniques to make your components more maintainable and scalable.
Understanding Props in Vue:
Props are a fundamental concept in Vue.js that allow data to be passed from a parent component to a child component. They enable the creation of reusable and customizable components by providing a way to configure component behavior from the outside. In Vue 3, props can be used seamlessly within the Composition API to enhance component functionality.
Declaring Props in Composition API:
In the Composition API, declaring props is straightforward. You can use the defineProps
function to specify the props that a component accepts. This function returns an object containing the prop values, which can then be accessed within the component logic.
import { defineComponent, defineProps } from 'vue';
const MyComponent = defineComponent({
setup() {
const props = defineProps<{ message: string }>();
// Accessing props
console.log(props.message);
return {};
},
});
Validating Props:
Vue allows you to validate props to ensure they meet certain criteria. This is especially important for maintaining component reliability and preventing potential bugs. You can use the PropTypes
object to define prop types and enforce validation rules.
import { defineComponent, PropTypes } from 'vue';
const MyComponent = defineComponent({
props: {
message: {
type: String,
required: true,
validator: (value: string) => value.length <= 50,
},
count: PropTypes.number.def(0),
},
setup(props) {
// Accessing validated props
console.log(props.message);
console.log(props.count);
return {};
},
});
Handling Prop Changes:
When a prop value changes, you may need to reactively update your component's state or trigger specific actions. In the Composition API, you can use the watch
function to watch for prop changes and respond accordingly.
import { defineComponent, watch } from 'vue';
const MyComponent = defineComponent({
props: {
message: String,
},
setup(props) {
watch(() => props.message, (newValue, oldValue) => {
console.log('Prop "message" changed:', oldValue, '->', newValue);
// React to prop changes here
});
return {};
},
});
Default Prop Values:
You can provide default values for props using the default
property. This ensures that if a prop is not provided by the parent component, your component can still function correctly with the default value.
import { defineComponent } from 'vue';
const MyComponent = defineComponent({
props: {
message: {
type: String,
default: 'Hello, World!',
},
},
setup(props) {
console.log(props.message); // Output: 'Hello, World!'
return {};
},
});
Conclusion:
Handling props effectively is crucial for building maintainable and flexible Vue components. With Vue 3's Composition API, you have powerful tools at your disposal for declaring, validating, and reacting to prop changes within your components. By following best practices and leveraging these features, you can create more robust and reusable Vue applications. Experiment with these techniques in your projects to streamline your component development process and enhance code quality.