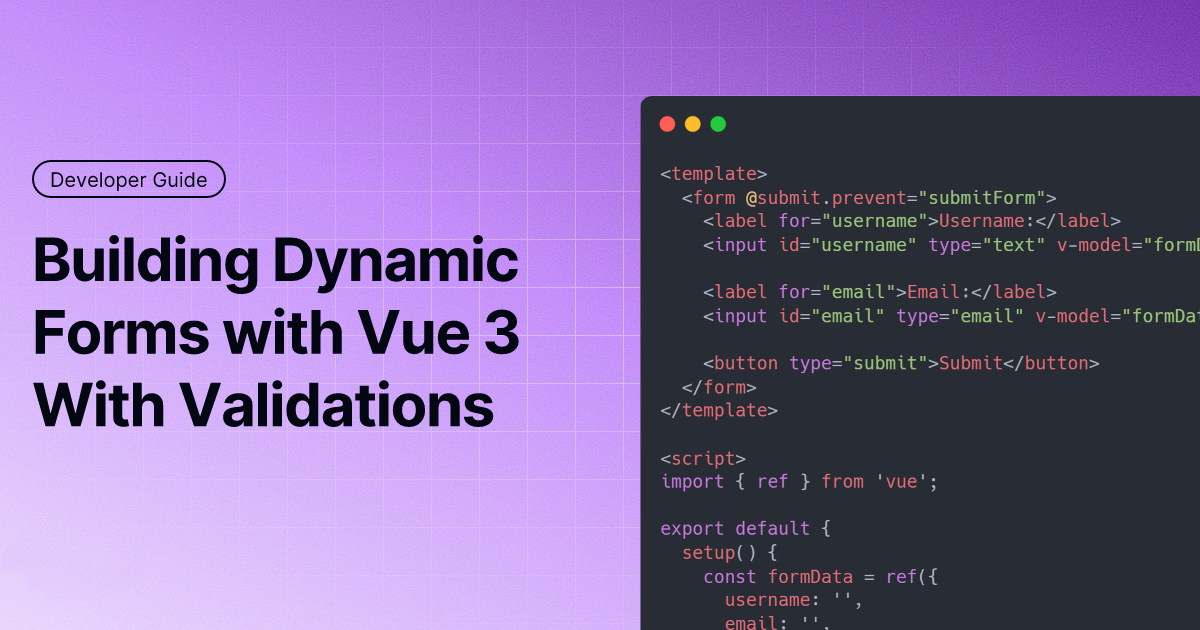
DEVELOPER GUIDEBuilding Dynamic Forms with Vue 3: Strategies for Handling Validation and User Interaction
Introduction:
Forms are a fundamental part of web applications, and Vue 3 provides powerful tools for building dynamic and interactive forms efficiently. In this blog post, we will explore strategies for building dynamic forms in Vue 3, focusing on handling form validation and user interactions. By leveraging Vue's reactivity system, component composition, and validation libraries, you can create robust and user-friendly forms that enhance the overall user experience.
- Form Data and Two-Way Binding:
In Vue 3, form data can be managed using reactive properties, such asref
orreactive
, combined with two-way binding using thev-model
directive. This allows for seamless synchronization between form inputs and data properties. Here's an example of a simple form setup in Vue 3:
<template>
<form @submit.prevent="submitForm">
<label for="username">Username:</label>
<input id="username" type="text" v-model="formData.username" />
<label for="email">Email:</label>
<input id="email" type="email" v-model="formData.email" />
<button type="submit">Submit</button>
</form>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const formData = ref({
username: '',
email: '',
});
const submitForm = () => {
// Handle form submission
console.log(formData.value);
};
return { formData, submitForm };
},
};
</script>
- Form Validation with VeeValidate:
Vue 3 offers various libraries for form validation, with VeeValidate being a popular choice. VeeValidate provides declarative validation rules and error messaging, making form validation intuitive and customizable. Here's an example of using VeeValidate for form validation in Vue 3:
npm install vee-validate@next
<template>
<form @submit.prevent="submitForm">
<label for="username">Username:</label>
<input id="username" type="text" v-model="formData.username" />
<span v-if="errors.username">{{ errors.username[0] }}</span>
<label for="email">Email:</label>
<input id="email" type="email" v-model="formData.email" />
<span v-if="errors.email">{{ errors.email[0] }}</span>
<button type="submit">Submit</button>
</form>
</template>
<script>
import { ref } from 'vue';
import { useForm, useField } from 'vee-validate';
export default {
setup() {
const { formData, submitForm, errors } = useForm();
const { value: username, errorMessage: usernameError } = useField('username', 'required|alpha_num');
const { value: email, errorMessage: emailError } = useField('email', 'required|email');
return { formData, submitForm, errors, username, usernameError, email, emailError };
},
};
</script>
- Dynamic Form Fields and Conditional Rendering:
Vue 3's reactivity system enables dynamic form fields and conditional rendering based on user interactions or data changes. You can use reactive variables or computed properties to control the visibility or behavior of form elements dynamically. Here's an example of dynamic form fields in Vue 3:
<template>
<form @submit.prevent="submitForm">
<label for="country">Country:</label>
<select id="country" v-model="selectedCountry">
<option v-for="country in countries" :key="country.id" :value="country.id">{{ country.name }}</option>
</select>
<div v-if="selectedCountry === 'other'">
<label for="otherCountry">Other Country:</label>
<input id="otherCountry" type="text" v-model="formData.otherCountry" />
</div>
<button type="submit">Submit</button>
</form>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const formData = ref({
selectedCountry: '',
otherCountry: '',
});
const countries = [
{ id: 'us', name: 'United States' },
{ id: 'ca', name: 'Canada' },
{ id: 'other', name: 'Other' },
];
const submitForm = () => {
// Handle form submission
console.log(formData.value);
};
return { formData, countries, submitForm };
},
};
</script>
Conclusion:
Building dynamic forms in Vue 3 involves leveraging Vue's reactivity system, two-way data binding, validation libraries like VeeValidate, and dynamic form field rendering techniques. By following these strategies and incorporating user-friendly interfaces, error handling, and submission logic, you can create highly interactive and robust forms that enhance user experience and ensure data integrity in your Vue.js applications. Experiment with these techniques and explore additional form management libraries to suit your specific project requirements and user needs.